Allow retries
Enable retry buttons
In your review template, you can enable retries in the settings of most field types like text, images, or markdown.
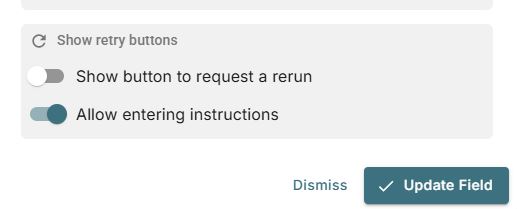
You can enable a simple retry button, or the option to enter instructions, or both.
Review UI
In the review UI, this will add corresponding retry buttons next to the field.
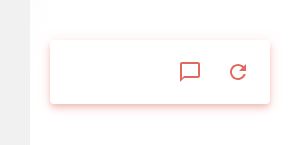
When a reviewer selects a retry option, a new button appears to send the instructions instead of submitting the review.
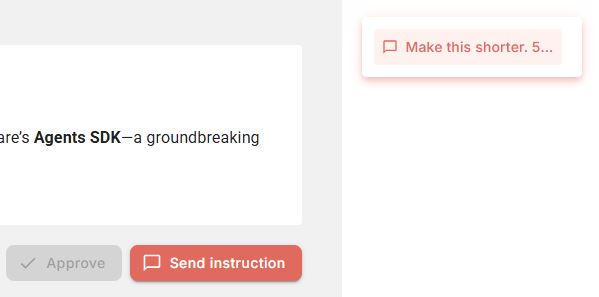
Webhook
Sending the instructions will trigger the webhook you set up for this review template with a "type": "instructions"
. The affected field will have an instruction
object, telling you how to rerun the step in your agentic workflow - simply running it again or inserting text instructions in your prompt template. Since it might be necessary to run multiple iterations/retries to reach a great result, for ease of use, it also incl. an allTextInstructions
array.
{
"type": "instructions",
"accountId": "aJ6A5ZqIeJjueTA76vv5",
"reviewId": "iVcm4fwRPihAdVnZ7v0d",
"formId": "ib41P20UBPLpEfwH7CkL",
"formName": "Review blog post",
"respondingUser": "[email protected]",
"respondedAt": "2025-05-05T16:15:53.000Z",
"responseValues": {
...
"blog": {
"value": "...",
"wasEdited": false,
"instruction": {
"type": "textInstruction",
"text": "Make this shorter. 500 chars max"
},
"allTextInstructions": [
"Make this shorter. 500 chars max"
]
},
"imageOptions": {
"value": [
{
"url": "https://cdn.example.com/my-image.jpg"
},
{
"url": "https://cdn.example.com/my-image2.jpg"
}
],
"instruction": {
"type": "rerun"
},
"allTextInstructions": []
}
}
}
Update review request
When a reviewer requests a retry or changes, the review changes to a waiting state. Your agent or workflow can now process the reviewer's instructions found in the webhook. When a new result is generated, you can update the review request. For simplicity you can send the same request as for your inital request for review, only adding the ID of the review to update.
- Python SDK
- JS/TS SDK
- HTTP
- Make
review = gotoHuman.create_review("ib41P20UBPLpEfwH7CkL") // ID of form/review template
review.update_for_review("iVcm4fwRPihAdVnZ7v0d") // <-- ID of the review
review.add_field_data("blog", "My blog post regenerated by the LLM to be shorter.")
review.add_field_data("imageOptions", [
{
"url": "https://cdn.example.com/my-regenerated-image3.jpg"
},
{
"url": "https://cdn.example.com/my-regenerated-image4.jpg"
}
])
// add other fields
try:
response = review.send_request()
print("Review sent successfully:", response)
except Exception as e:
print("An error occurred:", e)
Create a request with the formId of the form you created.
Pass the field values and optionally some meta data.
const reviewRequest = gotoHuman.createReview("ib41P20UBPLpEfwH7CkL") // ID of form/review template
.updateForReview("iVcm4fwRPihAdVnZ7v0d") // <-- ID of the review
.addFieldData("blog", "My blog post regenerated by the LLM to be shorter.")
.addFieldData("imageOptions", [
{
"url": "https://cdn.example.com/my-regenerated-image3.jpg"
},
{
"url": "https://cdn.example.com/my-regenerated-image4.jpg"
}
])
// add other fields
await reviewRequest.sendRequest()
The structure of your API request is
{
"formId": "ib41P20UBPLpEfwH7CkL", // ID of form/review template
"updateForReviewId": "iVcm4fwRPihAdVnZ7v0d", // <-- ID of the review
"fields": {
"blog": "My blog post regenerated by the LLM to be shorter.",
"imageOptions": [
{
"url": "https://cdn.example.com/my-regenerated-image3.jpg"
},
{
"url": "https://cdn.example.com/my-regenerated-image4.jpg"
}
],
// add other fields
}
}
Select Show advanced settings to show the field Update for Review ID, where you can enter or map the ID of the review to update.
Read more in our Make integration guide.